Today, I’ll guide you through building a QR code generator using HTML, CSS, and JavaScript. Create and customize QR codes easily.
Creating a QR Code Generator Using HTML, CSS, and JavaScript
In the digital age, Quick Response (QR) codes have become a ubiquitous part of our lives. They’re used for everything from sharing website links to contact information, and they offer a convenient way to access information with a simple scan. If you’ve ever wondered how to create your own QR code generator, you’re in the right place. In this tutorial, we’ll guide you through the process of building a QR code generator using HTML, CSS, and JavaScript.
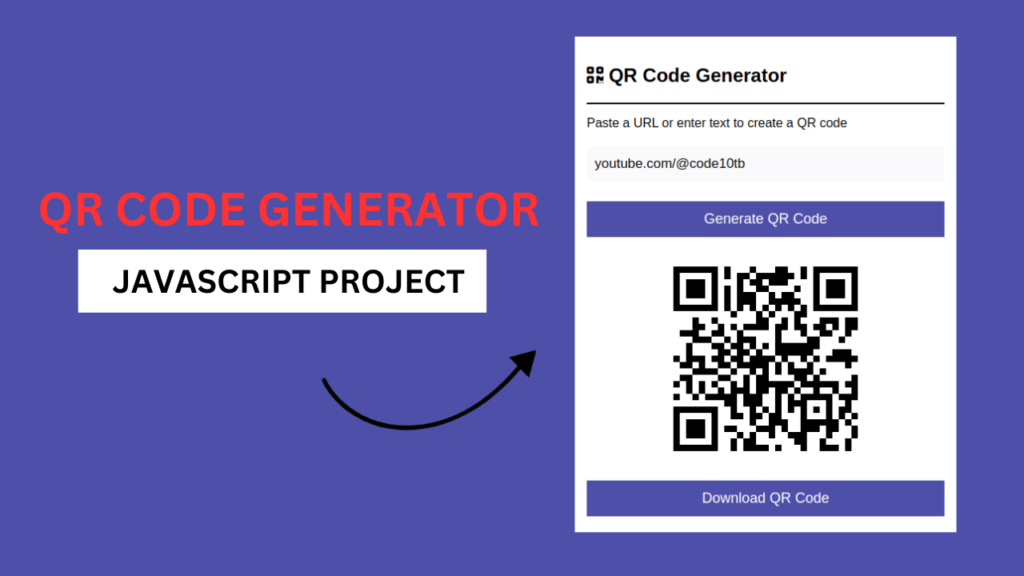
Setting Up the HTML Structure
We begin by setting up the basic HTML structure for our QR code generator. The HTML code defines the structure of the web page and includes the necessary elements, such as input fields and buttons. We’ve used a clean and minimalistic design to ensure a user-friendly experience.
Styling with CSS
To make our QR code generator visually appealing, we’ve added CSS styles. These styles set the color scheme, font choices, and overall layout of the web page. The styling is designed to create a pleasing and user-friendly interface.
The QR Code Generation Logic
Now, let’s dive into the JavaScript part of the code. We’ve included a QR code generation library called ‘qrcode-generator’ to create QR codes dynamically based on the user’s input. When a user enters text or a URL and clicks the “Generate QR Code” button, the JavaScript code takes the input, generates a QR code, and displays it on the page.
Download the QR Code
Once the QR code is generated, users can also download it. When the “Download QR Code” button is clicked, it triggers a download of the generated QR code as a PNG image file. This feature enhances the practicality of the QR code generator, as users can easily save and share the codes they create.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″ />
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″ />
<title>QR Code Generator</title>
<link
rel=”stylesheet”
href=”https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css”
/>
<style>
/* Reset styles and set default font-family */
* {
font-family: Roboto, sans-serif;
padding: 0;
margin: 0;
box-sizing: border-box;
cursor: pointer;
}
/* Styling for the body */
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #282a36;
}
/* Styling for the QR code wrapper */
.qr-code-wrapper {
width: 480px;
height: auto;
background-color: #44475a;
padding: 15px;
}
/* Styling for the QR code heading */
.qr-heading {
margin: 20px 0;
color: #bd93f9;
}
/* Styling for the horizontal rule */
hr {
height: 2px;
outline: none;
background-color: #f8f8f2;
border-style: none;
}
/* Styling for the text or URL input field */
.text-or-url {
font-size: 16px;
margin: 15px 0;
font-weight: 500;
color: #f8f8f2;
}
/* Styling for the input text field */
#text {
padding: 12px 10px;
width: 100%;
margin: 5px 0 20px 0;
outline: none;
border: none;
font-size: 17px;
background-color: #44475a;
color: #f8f8f2;
}
/* Styling for buttons */
.btn {
padding: 12px 10px;
width: 100%;
margin: 5px 0;
outline: none;
border: none;
font-size: 18px;
}
/* Styling for the “Generate” button */
.btn-generate {
background-color: #bd93f9;
color: #282a36;
}
/* Styling for the “Download” button */
.btn-download {
text-decoration: none;
background-color: #bd93f9;
text-align: center;
color: #282a36;
}
/* Styling for the QR code container */
#qrcode {
display: flex;
justify-content: center;
align-items: center;
background-color: #44475a;
border-radius: 2px;
margin: 10px 0px;
}
</style>
</head>
<body>
<div class=”qr-code-wrapper”>
<div>
<h2 class=”qr-heading”>
<span><i class=”fa-solid fa-qrcode”></i></span> QR Code Generator
</h2>
</div>
<hr />
<div class=”generator”>
<p class=”text-or-url”>Paste a URL or enter text to create a QR code</p>
<input type=”text” id=”text” placeholder=”Enter text or URL” />
<br />
<button id=”generate” class=”btn btn-generate”>Generate QR Code</button>
<br />
<div id=”qrcode”></div>
<a
id=”download”
download=”qrcode.png”
style=”display: none”
class=”btn btn-download”
>Download QR Code</a
>
</div>
</div>
<script src=”https://cdn.jsdelivr.net/npm/qrcode-generator@1.4.1/qrcode.min.js”></script>
<script>
document
.getElementById(“generate”)
.addEventListener(“click”, function () {
const text = document.getElementById(“text”).value;
if (text) {
const typeNumber = 0;
const errorCorrectionLevel = “H”;
const qr = qrcode(typeNumber, errorCorrectionLevel);
qr.addData(text);
qr.make();
const qrCodeCanvas = qr.createImgTag(8); // The “8” specifies the pixel size of the QR code
// Display the QR code
const qrCodeDiv = document.getElementById(“qrcode”);
qrCodeDiv.innerHTML = qrCodeCanvas;
// Show and set download link
const downloadLink = document.getElementById(“download”);
downloadLink.href = qr.createDataURL(8);
downloadLink.style.display = “block”;
} else {
alert(“Please enter some text or URL.”);
}
});
</script>
</body>
</html>
User Experience
We’ve put effort into creating an intuitive and user-friendly experience. Users are provided with clear instructions and input fields, making it easy for anyone to generate a QR code for their needs.
Further Customization
If you want to enhance this QR code generator further, you can customize the CSS styles to match your branding or project requirements. You can also add additional features, such as the ability to generate different types of QR codes (e.g., vCard, Wi-Fi, or email) or allow users to choose the QR code’s size and error correction level.
Dynamic QR Codes: You can extend the functionality of this generator to create different types of QR codes. For instance, you can allow users to generate vCard codes for contact information, Wi-Fi access codes, or even event-specific QR codes. This adaptability could make the generator even more versatile for various use cases.
Customization: Consider giving users the option to customize the QR code’s appearance, such as color, error correction levels, and logo integration. This added flexibility can cater to branding requirements and make the QR codes more visually appealing.
Data Analytics: For business applications, you can implement data analytics to track the usage and performance of generated QR codes. This valuable data can help you understand user behavior and the effectiveness of your QR code campaigns.
Mobile Optimization: Ensure that your QR code generator is mobile-responsive, allowing users to create and download QR codes from their smartphones or tablets. This mobile optimization can be essential, especially for on-the-go users.
Security Measures: Implement security features to prevent abuse, such as limiting the number of QR codes that can be generated within a specific time frame or CAPTCHA verification.
Integration: If you’re developing a website or application, you can integrate this QR code generator into your existing ecosystem, making it a seamless part of your digital platform.
In conclusion, a QR code generator built with HTML, CSS, and JavaScript offers a wide range of possibilities for both personal and business applications. It provides a user-friendly way to create QR codes, and with further customization and enhancements, it can be a valuable tool for various scenarios. Whether it’s for marketing campaigns, sharing contact information, or enhancing user engagement, this QR code generator serves as a solid foundation to build upon and adapt to your specific needs.
Conclusion
In this tutorial, we’ve demonstrated how to create a QR code generator using HTML, CSS, and JavaScript. This project allows you to generate QR codes for text or URLs with a simple user interface. You can use this code as a starting point and build upon it to create a more advanced QR code generator that suits your specific needs. Whether you’re building a personal project or integrating QR code generation into a business application, this tutorial provides you with the foundation to get started. QR codes are a powerful tool for sharing information, and with this generator, you can create them easily and efficiently. Watch the full video on YouTube click here